What is the DOM, exactly?
And why is it useful?
The Document Object Model, or DOM for short, is the data representation of the objects that make up the structure and content of a webpage document. The easiest way to interact with and view the DOM is to Right Click any webpage and select 'inspect', followed by selecting the 'Console' view.
From this Console view, we can write javascript functions to affect the rest of the webpage (Only temporarily, a refresh will remove and changed made). However, as a programmer using javascript, this view is most useful while writing a script; as a back-end language, it can be difficult to see if functions are working as they should. Therefore, using a console.log() to check if our code is actually creating change is a quick and easy way to avoid accidentally writing bad code that isn't properly connected to the HTML document.
Arrays and Objects
Storage Wars
Arrays and Objects are both used to store collections of data in javascript. The difference lies in how they each store this data.
Arrays store data in consecutive memory locations, which is a fancy way of saying each item is numbered, starting at 0. Arrays are useful when the items we wish to store have only one value, or that the order in which they are numbered also matters.
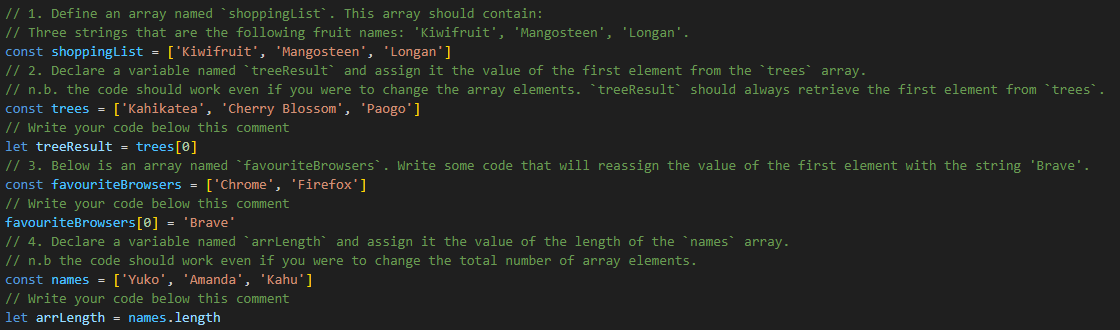
Objects store data in key-value pairs, so each key may hold more than one node of data. Objects are very useful when we need to assign more than one value to each item. During the creation of our Javascript cafe, each item on the menu is an object, with that item's stock and cost linked to each object.
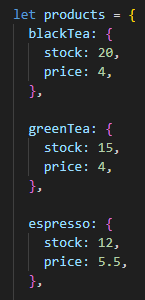
What are Functions?
What's their function?
A function is a code snippet that can be called by other code or itself. Arguments (values) are passed through the function as inputs, from which the function returns outputs. Below, we can see that we have 2 functions, within each are conditionals, variables, and loops.
When the function is called, the inputs are inputted, and the function runs everything through to give us the correct output.
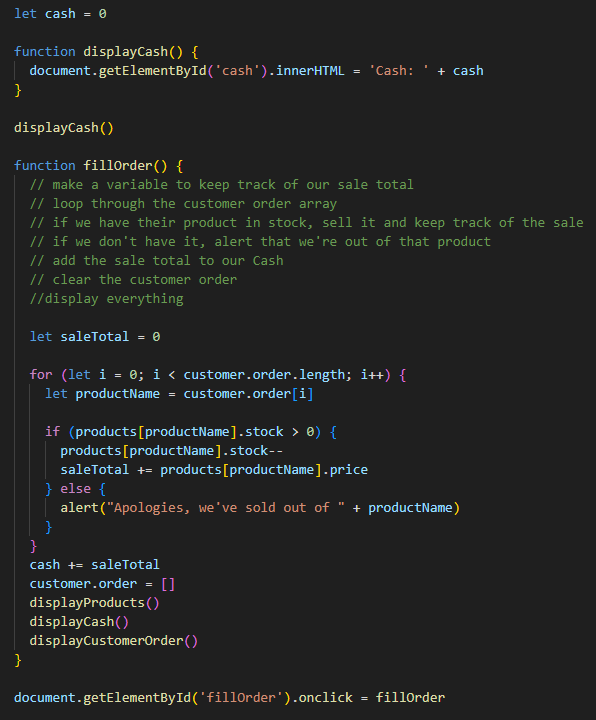